In this tutorial, We will learn about How To Share Text In Android Using Intents. But before we learn about that, let me give short introduction about intents for newbies.
What Are Intents ?
Intents allow you to interact with components from the same applications as well as with components from other applications.
For example, an activity can start an external activity for taking a picture using Intents.
An Intent is a simple message object that is used to communicate between android components such as activities, content providers, broadcast receivers and services. Intents are also used to transfer data between activities such as sharing text, images etc..
How To Share Text Using Intents
Android uses Intents and their associated extras to allow users to share information quickly and easily, using their favorite apps.
There are two ways for users to share data between apps in Android:
- The Android Sharesheet which is primarily designed for sending content outside your app and/or directly to another user. For example, sharing a Image or URL with a friend.
- The Android intent resolver which is best suited for passing data to the next stage of a some other task. For example, opening a Web URL in a web browser.
Here, we will use Android Sharesheet method for sharing Text. Sharing text is very easy in Android.
Following are the steps to share text in Android:-
- Create the intent class object for data.
- Set the ACTION_SEND as its action by setAction(Intent.ACTION_SEND) method. When you construct an intent, you must specify the action you want the intent to perform. Android uses the action ACTION_SEND to send data from one activity to another.
- Set the text you want to share using putExtra() method.
- Set the type of data which you want to send using setType(), here we are sending “text/plain” data.
- Then Finally, create shareIntent by using Intent.createChooser() method by passing the data Intent we created above to it and call startActivitymethod with this shareIntent.
MainActivity.java
package com.codingambitions.sharetext;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.support.v7.widget.AppCompatButton;
import android.view.View;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
AppCompatButton appCompatButton = findViewById(R.id.btn);
TextView textView = findViewById(R.id.text);
final String textToShare = textView.getText().toString();
appCompatButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent sendIntent = new Intent();
sendIntent.setAction(Intent.ACTION_SEND);
sendIntent.putExtra(Intent.EXTRA_TEXT, textToShare);
sendIntent.setType("text/plain");
Intent shareIntent = Intent.createChooser(sendIntent, null);
startActivity(shareIntent);
}
});
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<RelativeLayout
android:padding="16dp"
android:layout_width="match_parent"
android:layout_height="match_parent">
<RelativeLayout
android:layout_marginTop="32dp"
android:padding="16dp"
android:layout_centerHorizontal="true"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:textSize="18dp"
android:layout_centerHorizontal="true"
android:id="@+id/text"
android:layout_width="wrap_content"
android:layout_height="50dp"
android:text="@string/share_text_heading"
/>
<android.support.v7.widget.AppCompatButton
android:layout_marginTop="16dp"
android:background="@color/colorPrimary"
android:textColor="@color/white"
android:layout_below="@+id/text"
android:layout_centerHorizontal="true"
android:id="@+id/btn"
android:gravity="center"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="@string/share_button_text"/>
</RelativeLayout>
</RelativeLayout>
</android.support.design.widget.CoordinatorLayout>
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.codingambitions.sharetext">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name="com.codingambitions.sharetext.MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
res/values/strings.xml
<resources>
<string name="app_name">ShareTextExample</string>
<string name="share_text_heading">Sharing My First Text In Android Using Intents!</string>
<string name="share_button_text">Share Text In Android</string>
</resources>
Final Output of The App
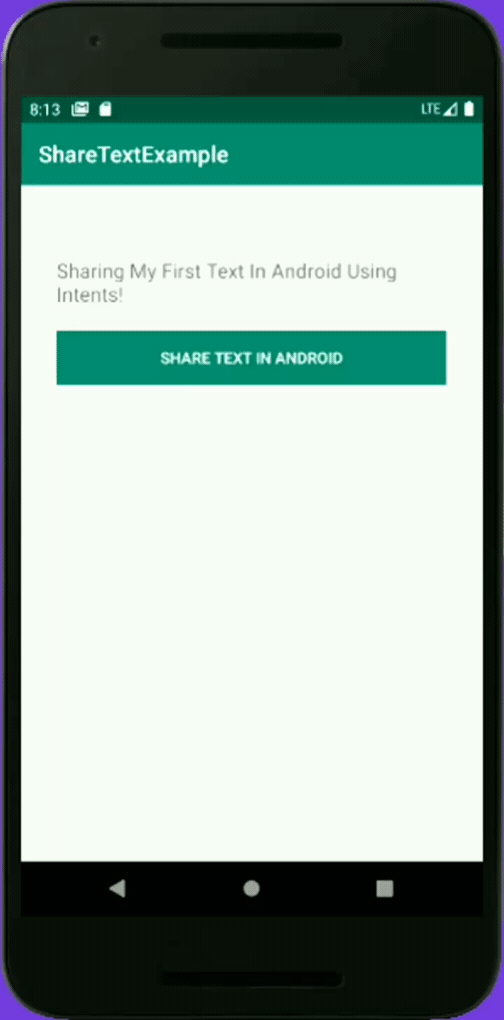
I hope you have understood this example on how to share text in Android. You can leave comments if you have any doubts.
Hurrah, you have created share Text app in Android.
Enjoy Coding!